How Child Classes and Inheritance work in JavaScript
An introduction to JavaScript classes, extends, and super
Hi y’all, I am back today with the second part of my introduction to JavaScript Classes.
Today, I am going to cover child classes, inheritance, the extends, and the super keywords. So, without further ado, let’s get this party started.
In my last post, I compared JavaScript classes to Chipotle. As a matter of fact, I said “I like to think of a class as being like Chipotle; people go in, and they come out with burritos.” Well as you probably know, you are not limited to burritos at Chipotle. You can also get a burrito bowl, tacos, or a taco salad, but all of those meals still have the same options as a burrito when it comes to ingredients.

So, to revise my previous statement, I like to think of a “parent class” as being like Chipotle; people go in, and they come out with meals. What about those meals, though? They may be different from one another, but they are made out of the same ingredients.

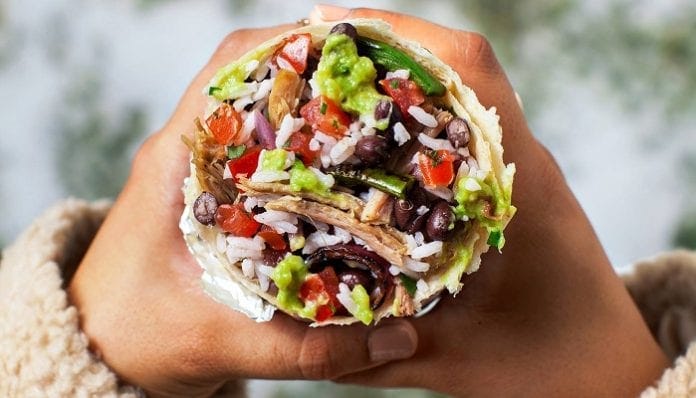
In order to create a Parent class, the first thing that we need to consider is what all of the meals all have in common. Whether you are getting a burrito, tacos, a burrito bowl, or a taco salad, they all share the following options: lettuce, beans, protein, veggies, cheese, guac, sour cream, and salsa….right? Since you can make a taco salad without adding to those ingredients, let’s make our parent class a taco salad.
Step 1: Class declaration

Now that we have the TacoSalad class declared, we want to add the constructor method. Remember, the constructor method is like your burrito artist (in this case your meal artist), which means that we need to give it the parameters of all the ingredients to make a taco salad, like so.
Step 2: Add a constructor & methods

Now we can make a salad with any ingredients that we choose, that wasn’t so bad, right? But, what if a customer comes in and they want a burrito bowl? We could create a new class called BurritoBowl, but a burrito bowl and a taco salad seem pretty similar. That would be kind of like having an ingredient station for taco salads, one for burrito bowls, one for tacos, and one for burritos.
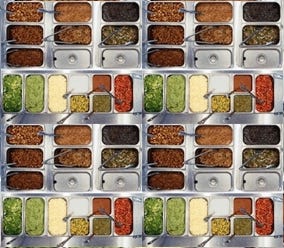
That would be pretty redundant right? Instead we could add rice to the ingredient station and add it to the meal only when it’s needed. This would be a good opportunity to use the extends keyword; we can use extends to create a class that is a child class of TacoSalad. The child class will inherit all of the key-value pairs and methods of the parent…kinda like I inherited my dad’s hairline. Let’s take a look at how this works.
Step 1: Class declaration with extends

Now that we have declared our class of BurritoBowl, we need to give it a constructor method just like we did with our TacoSalad class, but what are we going to put in the constructor? To me, it looks like a burrito bowl has the same ingredients as a taco salad except it is on a bed of rice, so let’s add a riceType parameter to our constructor.
Step 2: Add a constructor method

At this point, if we instantiate a new instance of BurritoBowl we will get an error because we are extending the TacoSalad class but we are not using the super keyword. A constructor can use the super keyword to call the constructor of the parent(or “super”) class allowing the child class to inherit all of the key-value pairs and methods from its parent. Wow, that keyword really is super! …and it’s going to save us a lot of code. Let’s see how it works.
Step 3: Add the super keyword

Let’s create a new instance a BurritoBowl to see how it works! Notice that since our BurritoBowl accepts one extra argument of riceType we will include that in the BurritoBowl constructor, however, since TacoSalad does not accept that argument we will leave it out of the super like so.
Step 4: Instantiating instances of a class
//add more to the fajita veggies array

Now, If we log newMeal (our new instance of BurritoBowl) you can see that it has inherited the key-value pairs from TacoSalad, and it has a riceType property as well. We can also log BurritoBowl.smellDelicious() and see that it has also inherited its methods from TacoSalad.




Now that you know how to create a child class, let’s create a Taco and Buritto class. Before you scroll down, go ahead and give it a shot. If you are feeling pretty confident, try adding a new method to the Taco class.

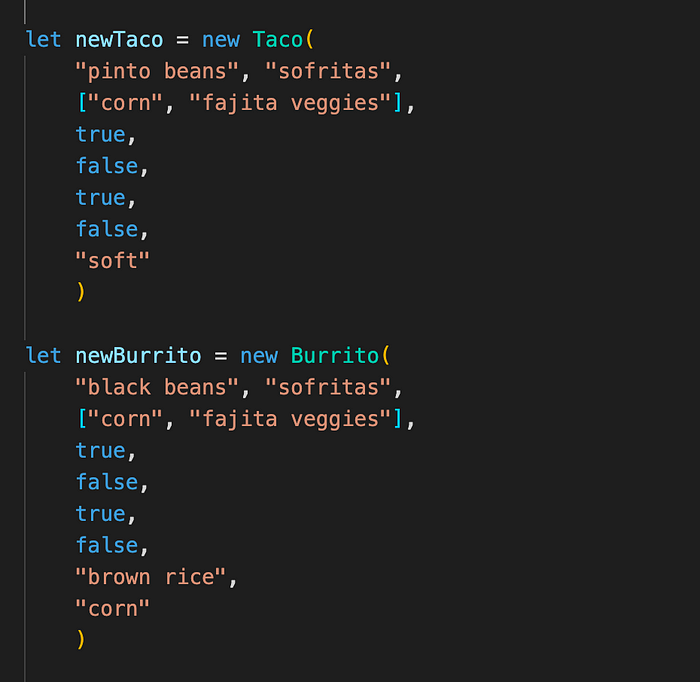
Going forward if we decide to add new items to our menu, we can use the extends and super key-words to create a child class, rather than duplicating code.
In this blog post we covered how to use inheritance along with the extends and super keywords to create child classes. We also covered the advantages of using child classes to make clean, reusable code. I hope that you found this article helpful; if you have any topics that you would like me to cover in the future, drop them in the comments.