JavaScript’s Destructuring Assignment
A Quick Way to Unpack Data
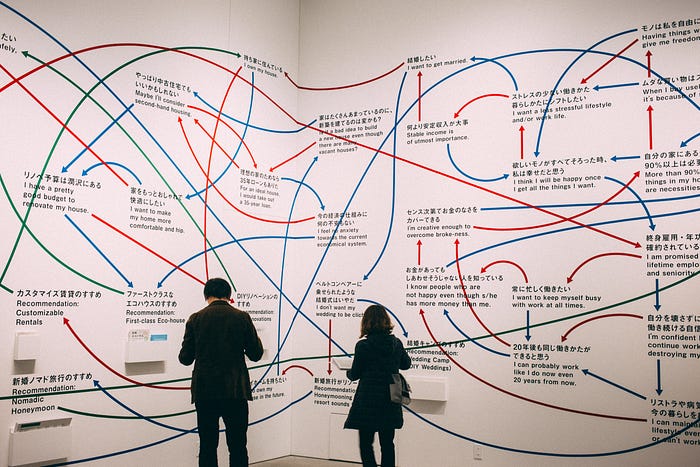
The destructuring assignment in JavaScript is a fast and easy way to delegate data from object and array literals to variables. If you ever find yourself writing line after line of variable assignments, consider using the destructuring assignment to save lines and time.
Basics of destructuring
Order is the name of the game when it comes to the destructuring assignment. The order of variables on the left side of the equality operator determines the values that will be assigned to them. If you remember nothing else about destructuring, remember this nugget. ORDER. MATTERS.
Syntax
For array destructuring:
[var1, var2, etc.] = [value1, value2, etc.]
For object destructuring:
( {var1, var2, etc.} = {key1: value1, key2: value2, etc.} )
***parenthesis are necessary for variable declaration only***
How to use
When assigning variables using the destructuring assignment, they must be wrapped in []
, otherwise only the last variable will be assigned to the values on the right of the equality operator. You can either declare the variables ahead of time or use variable assignment along with the destructuring assignment.
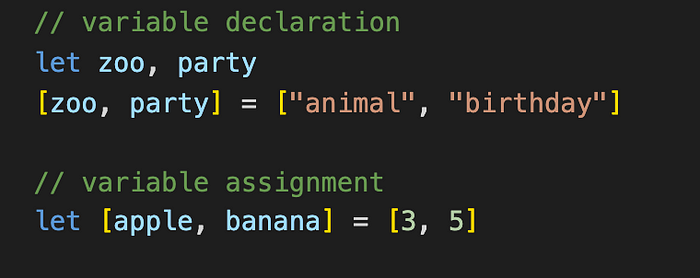
Let’s breakdown the code above to see what exactly is happening:
Variable declaration:
- Create two variables in memory called
zoo
andparty
. We won’t assign any values so they will remainundefined
. - Use the destructuring assignment to assign
zoo
to the first element in the array literal (aka"animal"
) andparty
to the second element (aka"birthday")
Variable assignment:
- Create two variables in memory,
apple
andbanana
, and immediately assign them to3
and5
, respectively.
We can also assign values to another variable, as we do with array
below, or to a function, such as newNums
, as long as they return an object or array literal.
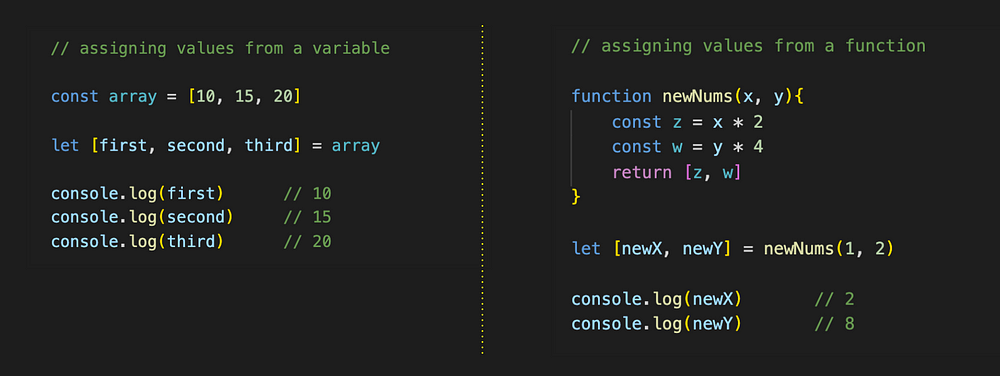
Assigning multiple values to a single variable
If you have fewer variables than values to assign, you can find yourself with “homeless” values. If you don’t need those extra values, then no problem, but if you, then we need a solution…

To retain all values, we can use the rest pattern to assign multiple elements in an object or array to a single variable. This pattern requires a spread operator, . . .
, followed by a variable of your choosing. When implementing this option, it must be the last variable in the destructuring assignment as it will assign all of the remaining values to it.

Skipping variables
Additionally, you can skip element assignments by adding blank
spaces in the destructuring assignment.

Assigning default values
If you have a set number of variables that need to be assigned a value, you can ensure that nothing returns undefined by assigning default values.
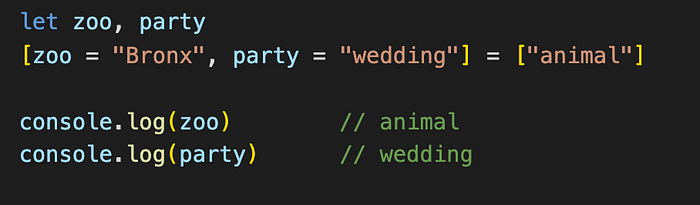
Looking at the code above, we see that zoo
has been given a default value of "Bronx"
while party
has a default value of "wedding"
. Because there is a value available for assignment, zoo
is now animal
. However, there is not a second value available for the variable party
so it retains its default value of "wedding"
.
Destructuring with objects
Destructuring objects is slightly different from destructuring arrays. In order for variables to be assigned new values from an object, they must have the same keys.
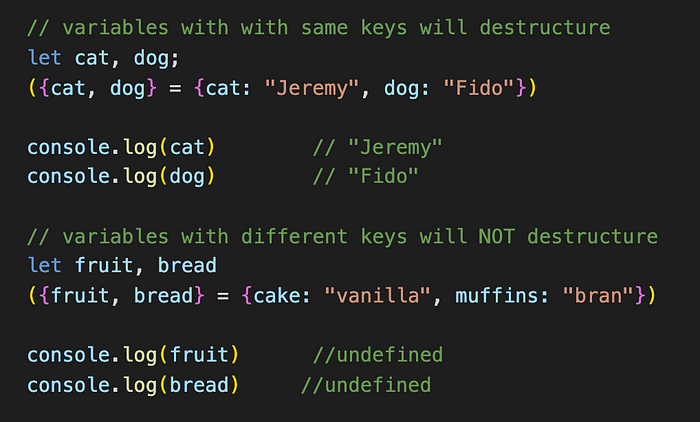
Additionally, object destructuring requires {}
around the variables on the left of the assignment operator. If you are using variable declaration, you will also need parenthesis()
around the entire destructuring statement.
More resources
If you are looking for more resources, the MDN web docs offer a great page on the destructuring assignment (link below). They go over the basics we’ve covered above as well more information on variable swapping, common errors, and destructuring a variety of different values like regular expressions and nested objects.