React Native full-screen notifications (even on Lock Screen)
Android Only

ALERT
As of android 10, SDK 29, This trick will no longer work for the device running Android 10, Because google has placed a restriction meaning, you won’t be able to start an application from the background.
Ref:
https://developer.android.com/guide/components/activities/background-starts
The Workaround for this would be to have a foreground service running in your application. You can take a look at the section for Exception for Restriction.
Here is a foreground service that i developed .
https://github.com/Raja0sama/rn-foreground-service
Note that foregorund solution is not tested i am assuming it since the docs mentioned that in the exception.
Well, you are probably here because you have been looking for a way to show a full-screen notification to the users of your react native app.
I have also been working on an app where the app is for the driver and android based and whenever an order is broadcast from the server driver should receive a notification, in my case a full-screen notification where he can answer whether he wants to accept the order or not. After a couple of hours of searching, I found no solution over the internet, which let you show a full-screen notification as it doesn’t exist.
Android has something called time-sensitive notification, which clearly states that.

In my case, I don't want it to show an intent which is a screen in normal words after the “interaction”, I want it to be a full-page notification, which it clearly does not support.
So What do we do now? How about we open the app on Notification Receiver and get some parameters before mount to navigate our app to a certain screen? In my case, What I did was created a standalone stack navigator with just one screen, connected it with firebase listener on mount displaying info and action buttons about the order.
Yes, That is all I need.
Then I started googling about opening activity on the notification receiver. Still, I found none, So opened up my Vscode and navigated to react-native-firebase/messaging and I found a file ReactNativeFirebaseMessagingReceiver.java where it has this function named onReceive where all the notification goes through, that's all I needed.
I started testing it; first I tried getting the data from remoteMessage like this
Log.d('Testing ',remoteMessage.getData().toString());
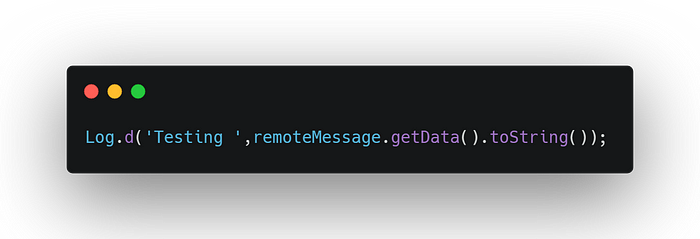
and in android Studio Log received was
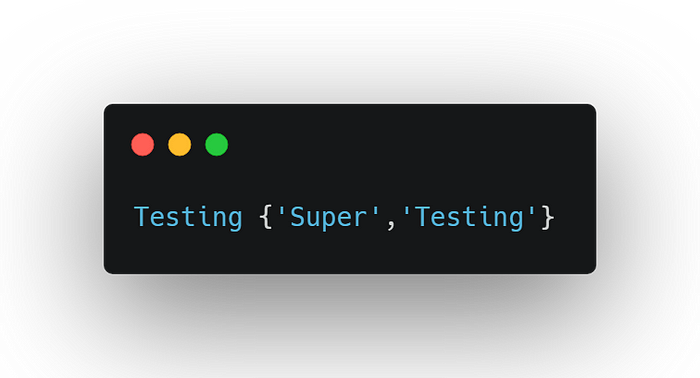
Bingo, I was receiving a map which is equivalent to javascript JSON Object,
I fetched Super next by using this line of code

I rebuild, and I got the Result Testing Testing. That's all I needed.
Next Step was to see if I can open up activity from the background, and as I was researching, I found many references that you can not open up activity from the background. So what to do now, Instead of trying to open an activity I tried opening an application, I tried to open my own application by mean of getLaunchIntentForPackage
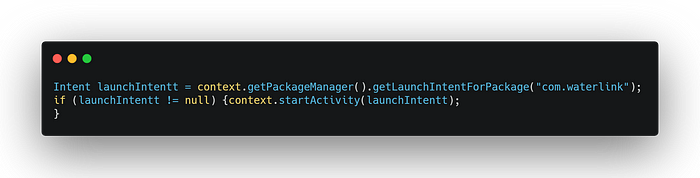
Bingo, onNotificationReceived if my application was in the background or killed because of push it was reopening my application which is all I ever wanted. On Active state, there was no different behaviour as the process I was trying to open already-opened; therefore, no change.
After that all of my problems were resolved, all of the problems were solved, and I put some condition checks on Data object to open up my application with props only where I need them to open like this.
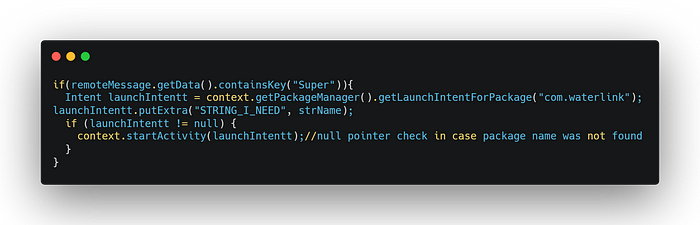
if (remoteMessage.getData().containsKey("Super")) {Intent launchIntentt = context.getPackageManager().getLaunchIntentForPackage("com.waterlink");
launchIntentt.putExtra("String_I_need", "strName");
if (launchIntentt != null) {
Log.d("Testing ", "Inside");
context.startActivity(launchIntentt);}
}
So far, everything was working; the last problem I faced was how to tell the app that the application is being opened by notification by not by a user.
There were two fixes, either I can send the data to the main activity utilizing putExtra, or I can have a listener on my app to check the order broadcast.
In my case, I went with the listener in my app, but for the put Extra you can easily solve it by using the piece of code in your mainactivity.java
go to your main Activity and declare a variable

and you need to override two methods of MainActivity.
Make sure you override onNewIntent First and then OnStart.
- onNewIntent
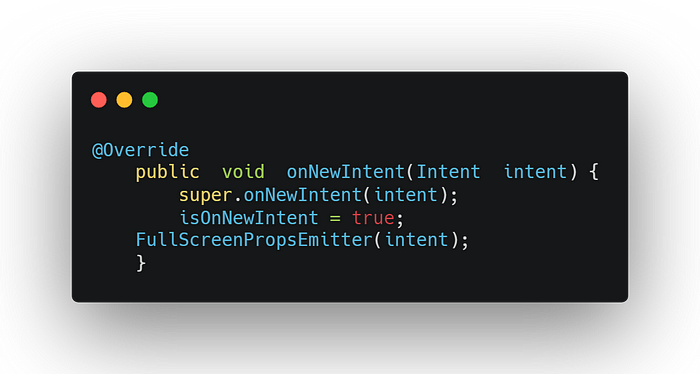
- onStart
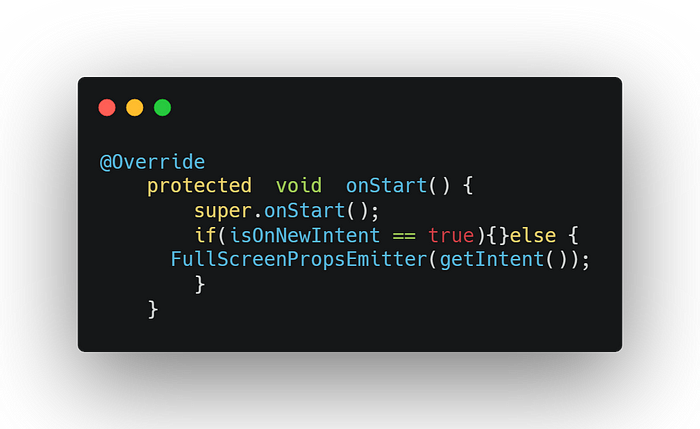
and in last our main Function — FullScreenPropsEmitter

and to fetch this props on your js
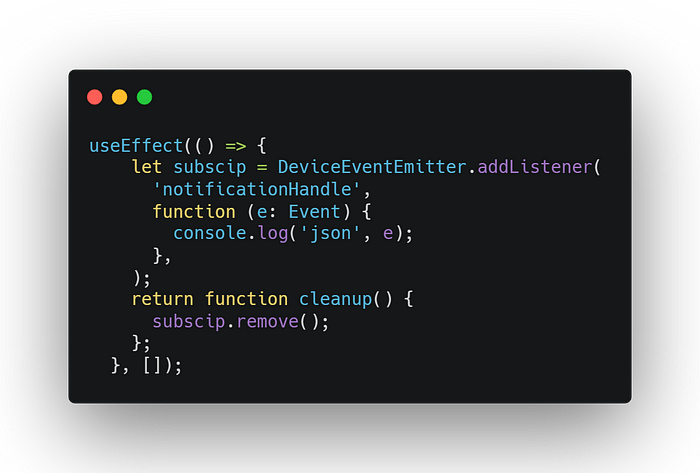
You can also use this method and send data as initial props by this method
And that is how you can open up you're react native app from firebase notification and send props with it and received props on the application start and show a specific screen for notification. I would recommend you to fork the library and use that in your app instead of using the official which will be overwritten if you yarn install again after deleting old node-modules.