13 Basic But Important JavaScript Interview Questions
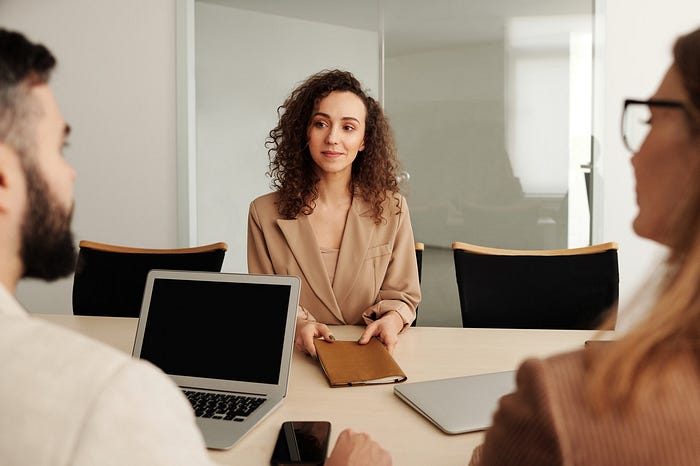
1. What is the difference between var, let & const variables?
- var — variables declared using var allow you to change the value of variables. Example: var a =13. Scope of variables declared using var is its current execution context and closures thereof, which is either the enclosing function and functions declared within it or, for variables declared outside any function, global.
- const — variables declared using const do not allow you to change the value once it is defined.
- let — variable may be reassigned value once it’s declared, such as a counter in a loop. It also specifies that the variable will be used only in the block it’s declared in.
2. What is the difference between == & === ?
The main difference between “==” and “===” operator is that the former compares variable by making type correction e.g. if you compare a number 1 with a string “1” with numeric literal “==” allows that & gives true value. “===” doesn’t allow that, because it checks the type of variables along with the value of two variables. If two variables are not of the same type “===” return false.
3. What is the difference between Null & Undefined?
Undefined means a variable has been declared but has not yet been assigned a value. On the other hand, null is an assignment value. It can be assigned to a variable as a representation of no value. Also, undefined and null are two distinct types: undefined is a type itself (undefined) while null is an object.
4. What will be the output when print null == undefined?
console.log(null == undefined)
true
console.log(null === undefined)
false
As defined earlier “==” only check the value & does not care about the type. If you print ‘===’ it gives false because undefined is not an object.
5. What is the difference between put & patch?
When a client needs to replace an existing Resource entirely, they can use PUT. When they’re doing a partial update, they can use HTTP PATCH. A PATCH is not necessarily idempotent, although it can be. Contrast this with PUT which is always idempotent. The word "idempotent" means that any number of repeated, identical requests will leave the resource in the same state.
6. What is the Strict mode in JavaScript and how can it be enabled?
Strict mode is a way to introduce better error-checking into your code. When you use strict mode, you cannot use implicitly declared variables, or assign a value to a read-only property, or add a property to an object that is not extensible. You can enable strict mode by adding “use strict” at the beginning of a file, a program, or a function.
7. What does the “this” keyword represent?
The ‘this’ keyword points to a particular object. Now, which is that object depends on how a function that includes 'this' keyword is being called. The following four rules applies to this
in order to know which object is referred to by this keyword.
- Global Scope
- Object’s Method
- call() or apply() method
- bind() method
8. What is object destructing?
The object destructuring makes it possible to unpack values from arrays, or properties from objects, into distinct variables.
9. What is CORS policy?
Most of the time, a script running in the user’s browser would only ever need to access resources on the same origin. So the fact that JavaScript can’t normally access resources on other origins is a good thing for security.
Cross-Origin Resource Sharing (CORS) is an HTTP-header-based security mechanism that is enforced by the browser which lets a server indicate any other origins (domain, scheme, or port) than its own from which a browser should permit the loading of resources. The Cross-Origin Resource Sharing standard works by adding new HTTP headers that let servers describe which origins are permitted to read that information from a web browser.
The browser uses pre-flight OPTIONS requests to check whether the HTTP method is allowed or not before sending the actual request to the server.
10. What are promises?
A promise is an object which either gives you a result or error in the future as soon as it completes the task. It is a popular design pattern used to handle asynchronous tasks in Node.js. It can take care of parallel/serial execution of many tasks along with their chaining rules. The promise structure also provides effective error-catching mechanisms. Learn more about promise here.
11. What is async await?
Async/Await is the extension of promises which we get as support in the language. async keyword, which you put in front of a function declaration to turn it into an async function. Invoking the async function returns a promise. This is one of the traits of async functions — their return values are guaranteed to be converted to promises. Learn more about async await here.
12. What is the event loop?
The event loop is what allows Node.js to perform non-blocking I/O operations despite the fact that JavaScript is single-threaded by offloading operations to the system kernel whenever possible. The event loop continuously checks the call stack to see if there’s any function that needs to run. The call stack is a LIFO (Last In, First Out) stack
13. What is the difference between forEach & the map function?
forEach executes a provided function once for each array element, while map creates a new array with the result of calling a provided fn on every element in the calling array. forEach mutates the calling array while map creates a new array.
Thanks for reading. Originally published on https://noob2geek.in on 13th June 2021.
More content at plainenglish.io