A brief introduction to Classes and Instances in JavaScript
Wu-Tang Clan + JavaScript Classes = Wu-Tang Class
When I first started working with JavaScript, I had a hard time understanding classes. I didn’t understand what they were, how they worked, or why you would use them; in this article, I am going to cover just that.
I like hip hop, so let’s say that we want to create an object that represents my favorite hip hop artist, the RZA from the Wu-Tang clan.
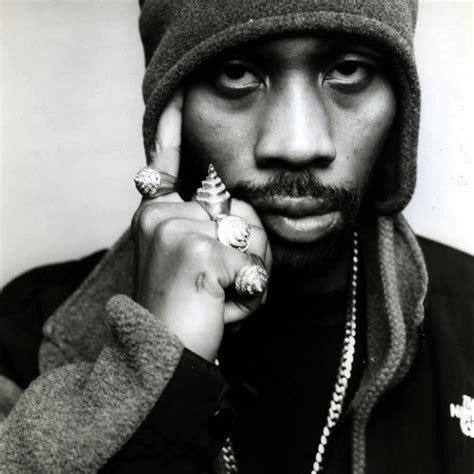
We could create an object literal that looks like this.
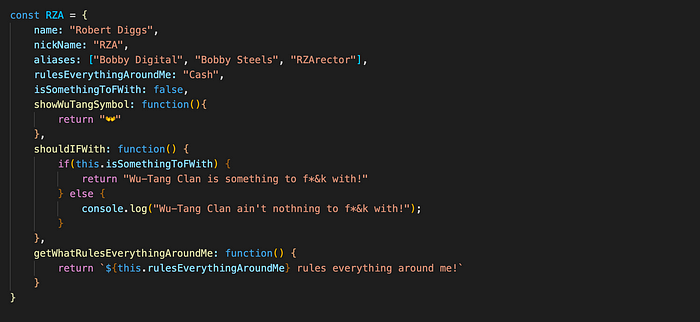
That’s pretty straightforward, right? Now, what if we want to make an object that represents each member of the Wu-Tang Clan?
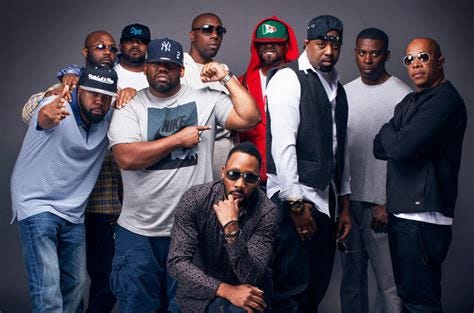
This might take a while. Now, this is where a JavaScript class would come in handy. A class is a basic template for creating objects in JavaScript. Each instance of a class will inherit key-value pairs and methods from the class. I like to think of a class as being like Chipotle; people go in, and they come out with burritos. What about those burritos, though? The burritos are different from one another, but they are still burritos. Let’s see how we can use a class of WuTangClanMember to create the rest of the members (rather than using object literals).
Step 1: Class declaration

Next, we will need to add a constructor method, the constructor() method is a special method that gets called when an instance of the WuTangClanMember class is created. I like to think of the constructor as the burrito artists at Chipotle. They accept certain parameters such as tortilla, rice, beans, protein, veggies, etc . If you give them the following arguments flour tortilla, brown rice, pinto beans, sofritas, and stir-fry veggies, you will get a burrito with those things in it. Now, let’s take a look at how this works with our WuTangClanMember class.
Step 2: Add a constructor method
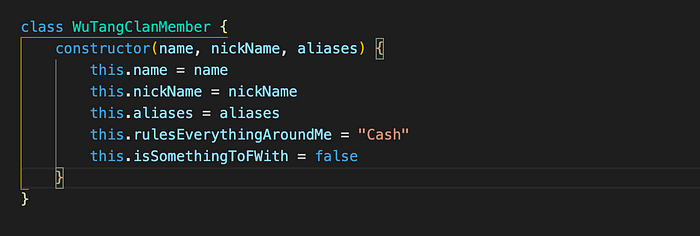
Now that we have our constructor set up, let’s look at how we can use methods with classes — after all, our objects would be boring if they didn’t do anything.
Step 3: Add methods

Now that we have our class of WuTangClanMember set up, we can use that to create instances of all of each Wu-Tang Clan member (This is also known as instantiating a WuTangClanMember). In order to create an instance of WuTangClanMember, we need to use the new keyword. Now, instead of creating an object literal for each Wu-Tang Clan member, we can instantiate instances of our “WuTangClanMember” class and save ourselves a lot of code.
Step 4: Instantiating instances of a class

Now, if you call the getWhatRulesEverythingAroundMe() method on each of our WuTangClanMembers, you can see that cash rules everything around each of them.


This has been a brief introduction to classes and instances in JavaScript. It doesn’t cover everything there is to know about classes, but hopefully, this article has shown you how to declare a class, how to use the constructor method, how to instantiate an instance of a class and the advantages of using classes over object literals. In my next post, the extends keyword to create child classes that will save even more time and code.