Uploading Files using Multer in Node.js Applications
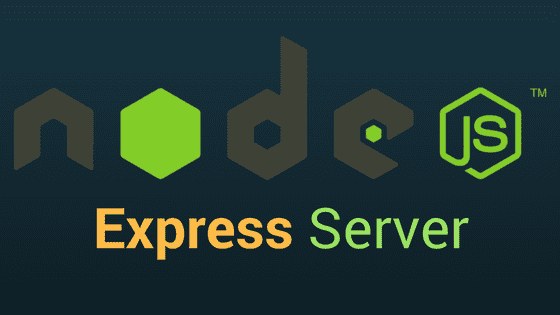
Table of Contents
Introduction
Today, we can find many apps and websites that upload images like photo sharing apps or blog websites. That is why, there are many good reasons to allow users store files on server. Http servers handle file uploading using POST requests. The case is different while talking about node.js. It is hard for node.js alone without using a library to handle uploading. That is the reason, there are many npm packages available. Multer is a good uploading npm package. It is mainly used with express.js.
Building web APIs is easy with Express.JS. I have already posted a tutorial about express.js. Multer is a npm package that handles multipart/form-data. It enables express.js applications to accept files. It is very easy to configure in express.js servers. This blog is minimized to teach only file uploading using multer, however it tries to give you a clue on how to link an image to a record in database and retrieve it later.
What We Build
In this tutorial, I am going to build a simple blog web API. It lets anyone write a blog which has title, content and photo. For that reason, I will use Express.JS to handle http requests, mongoose for storing data and Multer for uploading files.
Setup
- Create directories
mkdir file-upload
cd file-upload
- Initializing package.json
npm init -y
- Installing packages
npm i -S multer express mongoose body-parser uuid
npm i -D nodemon
- Start script: inside package.json, add a start script as bellow

- Creating express.js app with routes inside index.js file (entry file)
// import packages
const express = require("express");
const bodyParser = require("body-parser");
const mongoose = require("mongoose");
const path = require("path");// create and configure express app
const app = express();
app.use(bodyParser.json()); //parsing json files// routes
app.get("/blogs",
(req, res) => res.json({ success: true, blogs: [] }));
app.post("/blogs",
(req, res) => res.json({ success: true, blog: {} }));
app.put("/blogs/{id}",
(req, res) => res.json({ success: true, blogs: [] }));
app.delete("/blogs/{id}",
(req, res) => res.json({ success: true }));// this route is used for uploading
app.post("/blog/upload",
(req, res) => res.json({ success: true, blog: {} }));const PORT = 3000;
app.listen(PORT, (err) =>
err
? console.log(err)
: console.log(`The server is started on port: ${PORT}`)
);
Upload Images
Multer adds a
body
object and afile
orfiles
object to therequest
object.
If multer is configured to upload a single file then file property is added to the request field and if it is configured to upload multiple files files property is attached to the request object.
- Requiring NPM packages
const multer = require("multer");
const uuid4 = require("uuid").v4;
- Configuring storage
diskStorage is a type of configuration that is passed to multer and it is used to configure multer for storing files on disk permanently.
const storage = multer.diskStorage({
destination: path.join(__dirname, "/public/uploads"),
filename: function (req, file, cb) {
const fullName =
"blog_" + uuid4().replace(/-/g, "") +
path.extname(file.originalname);
cb(null, fullName);
},
});
const upload = multer({ storage: storage });
- Using multer as an express.js middleware.
app.post("/blogs/upload", upload.single("photo"), (req, res) =>
res.json({
success: true,
blog: {
photo: "/uploads/" + req.file.filename,
},
})
);
Things to Consider
- We need to allow express to serve public files, so we can be able to serve images uploaded.
app.use(express.static(path.join(__dirname, "public")));
- Multer can be further configured to handle the max file size limit to be uploaded. The fileSize is calculated in bytes.
- Files can be filters before uploading. There may be a case only images are allowed to be uploaded. Or the filter can be used for other things.
const upload = multer({
storage: storage,
limits: { fileSize: 2000000 },
fileFilter: function (req, file, cb) {
const fileTypes = /png|jpeg|jpg/;
const extName =
fileTypes.test(path.extname(file.originalname));
file.originalname.toLowerCase();
const mimeType = fileTypes.test(file.mimetype);
if (extName && mimeType) {
cb(null, true);
} else {
cb("Error: only png, jpeg, and jpg are allowed!");
}
},
});
An Example Demonstrating Multer
This example demonstrates file upload using multer for express.
Postman to Upload
For testing the API to upload a file, we are going to use postman.
- First paste the url “localhost:3000/blogs/upload/id_1234”
- Change the method to POST
- In Body tab choose form-data, and add key photo with type file.
- choose a file, then send the request.

A Complete Example
The bellow code is a complete example of file uploading and attaching to blog with four other different routing for retrieving, storing, updating and deleting a blog. It uses mongoose to connect to mongodb for reading and writing data.
Conclusion
Uploading files can be found in social networking applications, file hosting services like OneDrive and etc. In express.js applications, multer can easily be configured to handle file uploading.
You clone this repository for the complete code. I will be glad to hear from you, if you have any questions, concerns or suggestions.