Periodic Table of Elements in Svelte
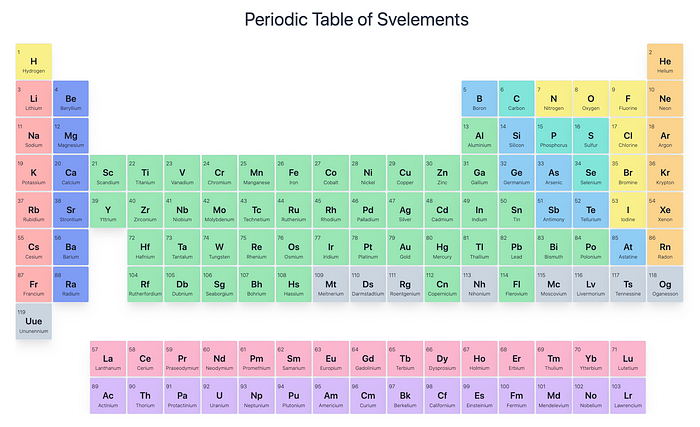
Recently, I’ve been playing around with Svelte
and I am amazed at how simple and easy it is to understand. There is also very little boilerplate code, so you can get right to coding with minimal headwind.
Working in a chemistry related industry, I use the periodic table of elements from time to time and wondered what it would be like to implement it in Svelte
. In this post, I share my journey in building the Periodic Table of Svelements
(Sorry, couldn’t help getting on the same bandwagon with all the Svelte
related puns!). Hopefully, this short tutorial can also serve as a brief introduction to Svelte
for those not so familiar.
Initial setup
For this project, I chose to use Svelte
with Tailwind CSS
, another awesome framework, to make it easier to style components. Tailwind CSS
is a utility first CSS framework that allows you to tweak the styling of your components by adding utility classes instead of using pre-styled components. If you are not familiar with Tailwind
, I suggest you check it out as well.
For the main project, an easy way to get started is to use the template at https://github.com/colinbate/svelte-ts-tailwind-template. The template includes Svelte
andTailwind
. It also includes support for TypeScript
, but we won’t use it in our project for now. You can jump start the template by heading in to your terminal
and following the steps below.
npx degit colinbate/svelte-ts-tailwind-template svelte-app
cd svelte-appnpm install
You can start the App using npm run dev
at the root directory. You can then go to localhost:5000
in your web browser. This will bring up a page with HELLO WORLD
.
In addition to the starter template, we also need some data with information about the elements. You can get a json
with all the required data from https://github.com/Bowserinator/Periodic-Table-JSON.
Next, create a folder called Data
inside your src
folder and put this json
inside. We will use this data to populate our periodic table.
There are many different methods for fetching json
data from a local file, one easy way is to use the rollup
plugin @rollup/plugin-json
. You can install this by following the steps below.
npm install @rollup/plugin-json
Then inside your rollup.config.js
file. Import the json
module and place it right under the import for typescript
.
import typescript from '@rollup/plugin-typescript';
import json from '@rollup/plugin-json';
Finally, in rollup.config.js
add json()
under plugins. You can place it below commonjs()
.
plugins: [
...
commonjs(),
json(),
...
]
Let’s get going
Looking at the periodic table of elements, we can see that elements are arranged in cells in a tabular format, hence the name. However, a table can also be thought of as a grid
, so we can probably use CSS grid
to achieve the same thing. I think I like that plan, so let’s go with it.
Let’s start by removing the unnecessary code in App.svelte
. Remove everything except for the imports for Tailwind CSS
. We can also remove the TypeScript
language support ( lang="ts"
) to keep things simple.
Finally, let’s add a header so that we can see something on the page. You should end up with the below code block. Running npm run dev
should bring up a page with the appropriately styled header. That’s it for the initial setup.
Creating individual elements
Before we create the table, let’s create our <Element />
component to make use of the data from the json
file.
Create a folder called Components
inside src
. Create a new file called Element.svelte
. Copy the code block below and allow me to explain what’s happening right after.
In our script
tag we do several things.
- Import and extract the
json
data into a variable calledelements
usingobject destructuring
. - We expose two props to our parent
component
(Table.svelte
, which we haven’t created yet). TheatomicNumber
prop will be used to pass in the appropriate atomic number, which our<Element />
component will use to retrieve the appropriate data from thejson
file. We also expose astyle
prop, which we can use to pass CSS styles like normal HTML. - We also create a function called
toCamelCase()
, which as the name suggests, converts a string into camelCase. Because the categories in thejson
file are strings with spaces, we use this to transform them, so that we can easily target them with CSS. This step is not necessary and is simply a preference.
In <style />
, we basically make our design responsive by using vw
as our unit of dimension. We also set colors for the different categories.
Finally, below style
is the markup for the <Element />
component. We setup each element as a button
. We then add styling via Tailwind's
utility classes. We access each element’s information by accessing them using their index (their atomic number less 1) in the elements
array. Properties like name
and symbol
are the property names from the json
.
Bringing the elements into the table
The next step is to bring our elements into our table (grid). Create a file called Table.svelte
inside theComponents
folder. Add the below code to the file.
We basically create our grid with 18 columns. Then we populate our grid with our <Element />
component. The next thing we need to figure out is how to position our elements according to the periodic table.
Fortunately for us, the json
data we imported at the start has all the information we need to achieve this. It has the atomic number of the element stored in the number
property and the x and y positions of each element stored in the xpos
and ypos
properties respectively.
We then use Svelte's
super handy {#each}
block to loop over our array of elements. We are almost done at this point. We only need to import our <Table />
component in our App.svelte
file.
Edit App.svelte
to look like the following.
Run npm run dev
, if you already haven’t and we’re done! You should have a responsive and slick Periodic Table of Svelements running locally at localhost:5000
.
You can find the completed project and all of the source code at https://github.com/kinxiel/Periodic-Table-of-Svelements.
At this point we have our periodic table but it doesn’t do anything useful. In part 2, we explore how to add some basic controls to our periodic table to display additional data.
Update: Part 2 is ready and you can find it at https://medium.com/javascript-in-plain-english/periodic-table-of-elements-in-svelte-part-2-98f3f7b2515